Why do we need both apps and projects in Django?
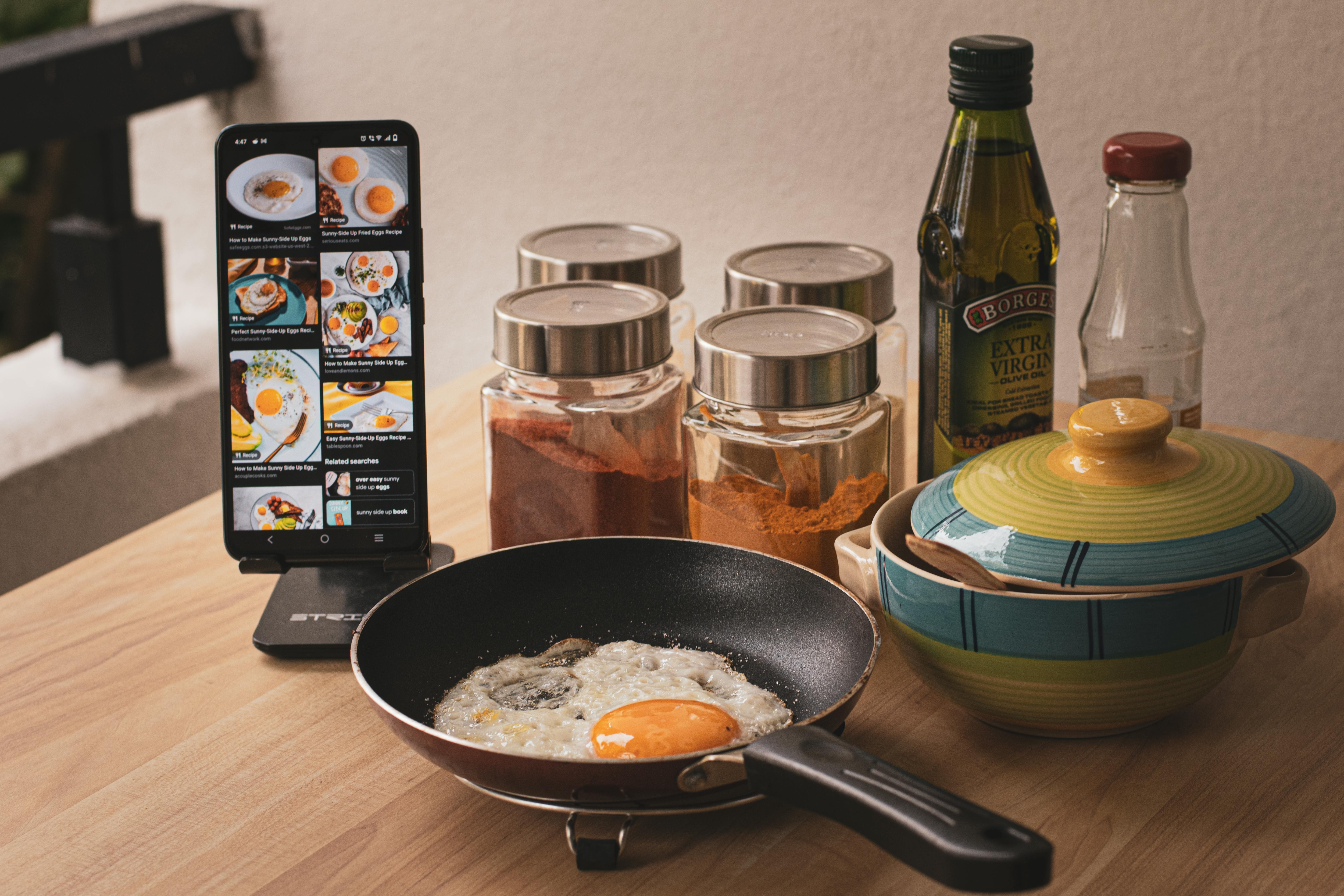
While continuing to follow the instructions here on setting up a new Django to-do list app, I got to this point in the instructions:
## Step 2: Creating a Django Project
Now, let's create a new Django project:
```bash
# Create a new Django project
django-admin startproject todo_project .
# Create a new app within the project
python manage.py startapp todo_app
```
I was confused about the distinction made in the comments about projects vs. apps in Django. After some digging, I discovered that the distinction between "apps" and "projects" is fundamental to Django's architecture.
According to Claude:
The distinction (between a project and an app) is that a Django "project" is the entire web application, while an "app" is a modular component within the project that handles specific functionality. A single project can contain multiple apps, and apps can be reused across different projects.
I asked Claude to further explain the concept of apps by giving me an example of a real-world project which contains multiple different apps. Its response:
Here's an example of a real-world e-commerce project with multiple Django apps:
E-commerce Platform Project
User Management App
- Handles user registration, authentication, profiles
- Manages user permissions and roles
- Implements password reset functionality and account settings
Product Catalog App
- Manages product listings, categories, and tags
- Handles inventory tracking
- Implements product search and filtering
- Manages product reviews and ratings
Shopping Cart App
- Handles shopping cart functionality
- Manages add/remove/update items
- Calculates totals, taxes, and discounts
- Saves carts between sessions
Checkout App
- Handles checkout process
- Processes payment information
- Handles shipping address collection
- Manages order creation and confirmation
- Integrates with payment gateways
Order Management App
- Tracks order status and history
- Handles order fulfillment workflows
- Manages shipment tracking
- Processes returns and refunds
...
This modular approach allows different teams to work on separate apps simultaneously, makes the codebase more maintainable, and allows for reusability across other projects if needed.
The above helped clarify the difference for me- I can easily picture an app with the above domain concepts, and it makes sense to separate the business logic cleanly so that it doesn't get muddled together.
At first I wasn't sure if there was a Rails equivalent to this separation of duties via apps. The closest thing I could think of was the concept of Rails controllers, but that didn't feel accurate. While controllers are typically split according to business logic, they're also specifically related to executing requests which get routed from the routes.rb file.
Out of curiosity, I then ask Claude if there is a similar concept in Rails to Django's "apps vs. projects" distinction. Its response:In Rails, there isn't an exact one-to-one equivalent to Django's "apps vs projects" distinction, but there are comparable concepts:
In Django:
- A project is the entire web application
- Apps are modular, reusable components within the project
- Each app handles a specific piece of functionality
In Rails:
- The closest equivalent to a Django "project" is simply a Rails application itself
- Instead of "apps," Rails uses several modular concepts:
- Engines: Self-contained mini-applications that can be mounted within a main Rails app (closest to Django apps)
- Concerns: Modules that encapsulate shared functionality across controllers or models
- Gems: Packaged Ruby libraries that can add functionality to Rails apps
The key differences:
- Rails applications are typically more monolithic, with a standard directory structure for models, views, and controllers
- Django encourages modularity from the start, with apps being self-contained
- Rails Engines are more complex to set up than Django apps and aren't used as frequently for internal organization
- Rails leans more heavily on namespacing within the application, while Django uses separate app directories
If you wanted to achieve the Django-style modularity in Rails, you'd typically use Engines for truly separable components or use namespaces/modules to organize related code within a single Rails application.
So to summarize, the closest Rails concept to Django's concept "apps vs. projects" distinction is that a Rails app is like a Django project, while a Rails engine is like a Django app.
After running the commands django-admin startproject todo_project .
and python manage.py startapp todo_app
, my virtual environment's main directory (~/Desktop/Workspace/Django/django_todo/venv
) looks like this:
$ ls -la
total 24
drwxr-xr-x 10 richiethomas staff 320 Mar 22 18:45 .
drwxr-xr-x 3 richiethomas staff 96 Mar 20 14:03 ..
-rw-r--r-- 1 richiethomas staff 69 Mar 20 14:03 .gitignore
drwxr-xr-x 14 richiethomas staff 448 Mar 20 20:47 bin
drwxr-xr-x 3 richiethomas staff 96 Mar 20 14:03 include
drwxr-xr-x 3 richiethomas staff 96 Mar 20 14:03 lib
-rwxr-xr-x 1 richiethomas staff 668 Mar 22 18:44 manage.py
-rw-r--r-- 1 richiethomas staff 403 Mar 20 14:03 pyvenv.cfg
drwxr-xr-x 9 richiethomas staff 288 Mar 22 18:45 todo_app
drwxr-xr-x 8 richiethomas staff 256 Mar 22 18:45 todo_project
Notice the two directories at the end: "todo_app/" and "todo_project/". The 1st directory (along with the file manage.py
) was created by the command python manage.py startapp todo_app
, and represents our first app. Conversely, the 2nd one was created by the command django-admin startproject todo_project .
, and represents the Django project as a whole.
Summary
An app is a self-contained module that provides a specific functionality, while a project is a collection of apps that work together to form a complete web application. This separation allows for better organization, reusability, and maintainability of code.
Photo Attribution
Title: Fried Egg on a Pan
Description: n/a
Author: Pranjall Kumar
Source: Pexels
License: Free To Use