Why do we need to create a virtual environment for Django projects, but not for Rails projects?
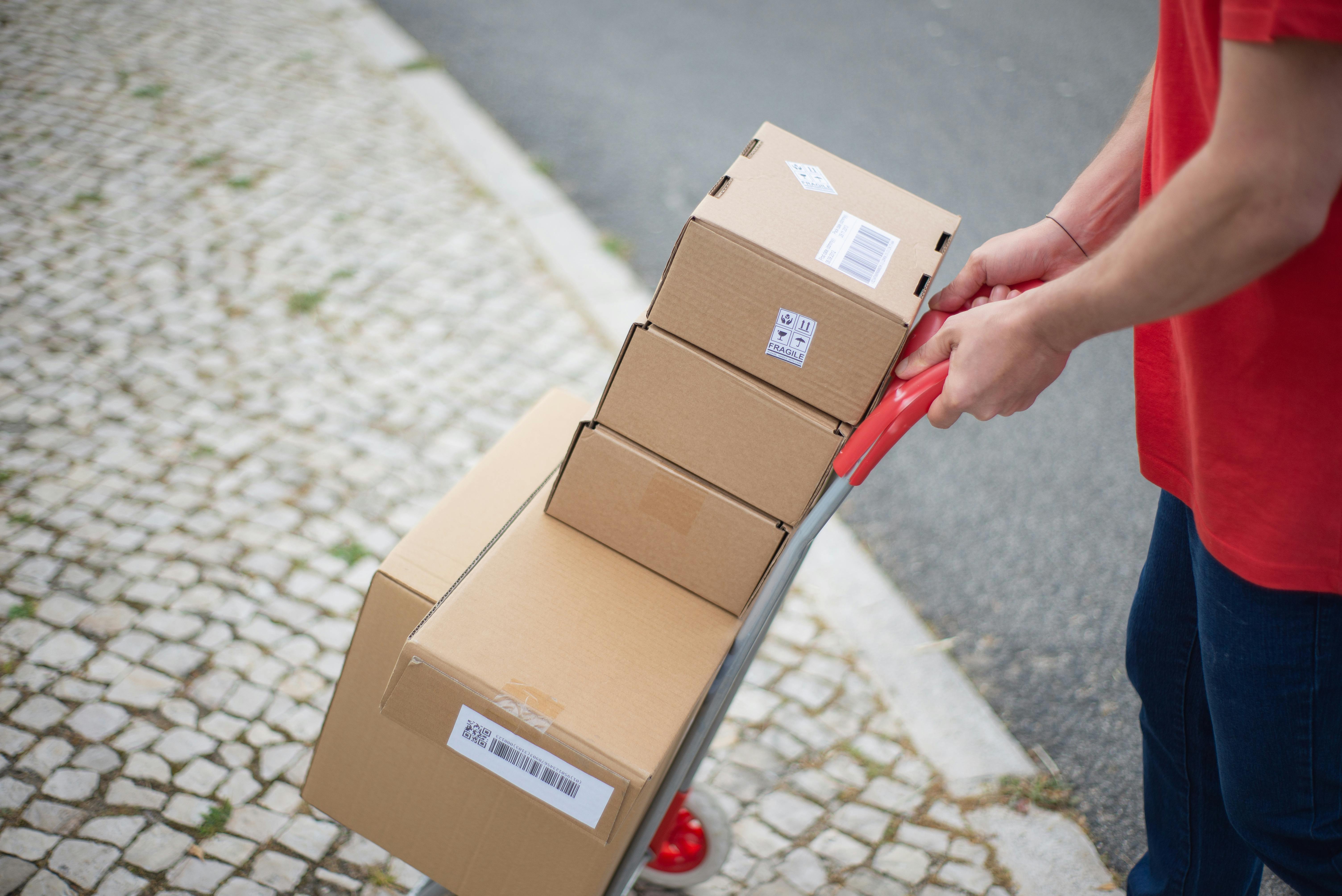
I'm starting to learn Django development now, as a side project while I do my job search. I've seen just as many Python/Django job postings (if not more) as I've seen Rails postings. And with AI becoming more and more common of a requirement in the engineering field, it feels prudent to have some exposure to Python in general.
One of the first things I did was ask Claude to help me set up a simple Django to-do app. After setting up the directory I'll be working in, the first few instructions are as follows:
# Create and activate a virtual environment python -m venv venv source venv/bin/activate # for Mac/Linux
The comment says the first line "create(s) and activate(s) a virtual environment". This is not something I've had to do (at least, not consciously) when building Rails apps. Side note- In Python, virtual environments aren't strictly required, but they're strongly recommended to avoid dependency conflicts.
In a separate Claude chat, I ask why this is necessary with Django, but not with Rails. The response I get is:
Django and Rails handle their dependencies and project isolation differently:
For Django (Python):
- Python lacks built-in project-specific dependency management
- Virtual environments (venv, virtualenv, conda) create isolated spaces where packages are installed only for that project
- Without them, all packages would be installed system-wide, risking version conflicts between projects
- Python's package manager (pip) doesn't have built-in project isolation
For Rails (Ruby):
Ruby has Bundler, which manages gems (Ruby packages) at the project level Bundler reads the Gemfile to install the correct versions of dependencies for each project Ruby's gemsets (especially with RVM or rbenv) provide isolation between projects Rails comes with this infrastructure built-in as part of its workflow
The key difference is that Rails has integrated dependency management through Bundler as part of its standard setup, while Django relies on Python's external virtual environment tools to achieve similar isolation.
What stood out to me first is the point about Python lacking built-in project-specific dependency management, so I did some digging. I discovered that, when you install dependencies inside a virtual environment, they are stored in a site-packages/
directory inside the virtual environment (e.g., venv/lib/python3.13/site-packages/
). This ensures that dependencies for one project don't interfere with system-wide Python packages or packages from other projects.
The virtual environment created for a Django project contains a directory called bin/
. This directory contains, among other things, several symlinks to the Python executable itself:
~/Desktop/Workspace/Django/django_todo/venv () $ ls -la bin/
...
-rw-r--r-- 1 richiethomas staff 2135 Mar 20 14:03 activate
...
lrwxr-xr-x 1 richiethomas staff 10 Mar 20 14:03 python -> python3.13
lrwxr-xr-x 1 richiethomas staff 10 Mar 20 14:03 python3 -> python3.13
lrwxr-xr-x 1 richiethomas staff 98 Mar 20 14:03 python3.13 -> /opt/homebrew/Cellar/python@3.13/3.13.0_1/Frameworks/Python.framework/Versions/3.13/bin/python3.13
...
Above, we can also see a file in the same bin/
directory called activate
. This is the file we're executing when we run source venv/bin/activate
as per Claude's instructions. This file updates our $PATH
variable inside the virtual environment to include the python
executables inside bin/
before any other executables, ensuring this is the executable which gets used when we run Python programs inside the virtual environment.
Conversely, in Ruby, dependency management is a bit different. If you're using a Ruby version manager, each version manager takes a different approach. For example, I use rbenv
to manage my Ruby versions. By default, RBENV stores each version of Ruby in a different folder, and stores gems for each Ruby version inside a sub-folder of that Ruby version's main folder. However, you can also explicitly install gems inside your project's directory structure (most commonly inside a folder named vendor/
).
With RBENV, gems are installed separately for each Ruby version. If you install a gem while using Ruby 3.0, switching to Ruby 3.1 (rbenv use 3.1) means that gem won't be available until you install it again for that version.
Separately, Ruby uses the concept of a Gemfile as a dependency manifest file for a given project, and a program called Bundler reads that Gemfile (or more specifically, the Gemfile.lock
file which specifies the versions of a project's gems) at runtime and modifies the Ruby load path to only include the specified gem versions for that project.
In summary, Ruby version managers like RBENV are in charge of managing both Ruby versions and the locations of each version's gems. Additionally, Bundler is in charge of including just the Ruby gem versions required for a given project. It's this one-two punch which allows Ruby projects to avoid storing their own dependencies inside the project folder.
Photo Attribution
Title: A Person Delivering a Boxes
Description: n/a
Author: Kampus Production
Source: Pexels
License: Free To Use