Class Attributes In Django
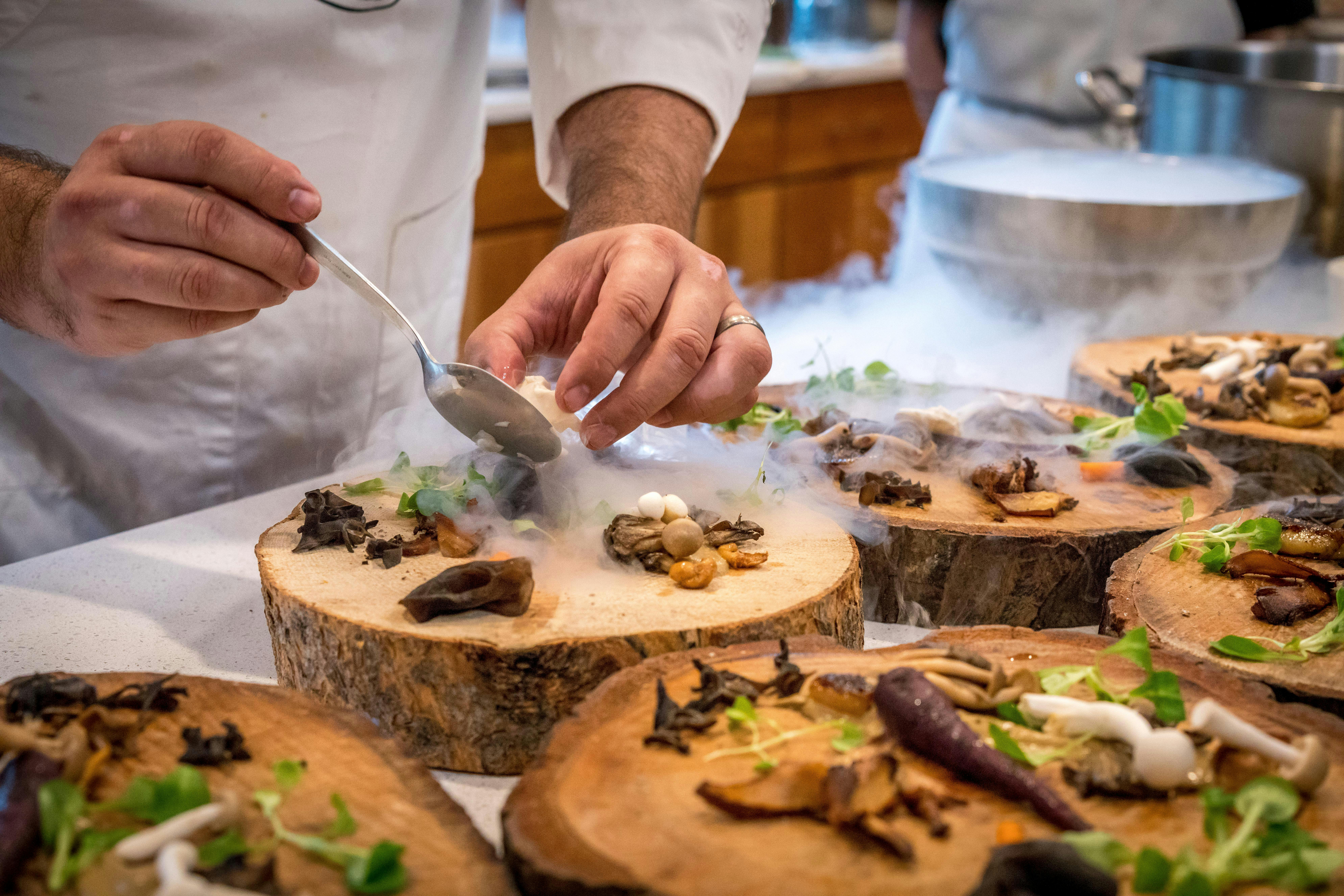
While making a simple to-do app in Django as part of a guide that ClaudeAI wrote for me, I came across the following code:
...
class TodoListView(ListView):
model = Todo
template_name = 'todo_app/todo_list.html'
context_object_name = 'todos'
...
I assumed (and ChatGPT confirmed) that this code creates a class named "TodoListView", which inherits from a more generic "ListView" parent class. So far, so good. However, the variable declarations in the body of the class look strange to me, especially since I come from a Ruby background.
It's as if we're declaring a class, and then declaring several local variables inside the class. That's a bit non-sensical, so I'm assuming that's not what this does, but I'm not sure what it *does* do. So I asked ChatGPT to explain. Its response:
...in Python (and Django), those look like regular variable assignments, but they are actually class attributes rather than instance attributes.
Key Difference from Rails:
In Ruby on Rails, you would typically define instance variables inside methods (e.g.,
@todos = Todo.all
in a controller action). However, in Django class-based views, attributes likemodel
,template_name
, andcontext_object_name
are class attributes, which Django'sListView
uses to configure the view.How This Works in Django:
ListView
is a Django generic class-based view (CBV).Django internally reads these class attributes and uses them to configure the view when handling a request.
Behind the scenes,
ListView
provides a method calledget_queryset()
, which retrieves the model data. You can override it if needed.Equivalent in Rails:
In a Rails controller, the equivalent would be:
class TodosController < ApplicationController def index @todos = Todo.all render "todos/index" end end
But in Django,
ListView
abstracts this logic, so you don't have to manually define a method to fetch records.
OK, so "class attributes" are just the Django equivalent of "class variables" in Ruby. Same with "instance attributes" vs. "instance variables". And instead of the Rails method of defining a `TodosController` class which would contain all of our controller actions (index
, show
, etc.), we define separate classes for each "action" for our business object, i.e. ToDo instances.
So does Django have a ShowView
parent class, which would be the equivalent of the Rails show
action? Or an EditView
class, which would equate to Rails' edit
action? Not quite, but close! According to ChatGPT, these are the Django parent classes, with their Rails equivalents:
Django View | Rails Equivalent |
---|---|
ListView | index (e.g., Todo.all ) |
DetailView | show (e.g., Todo.find(params[:id]) ) |
CreateView | new + create (e.g., Todo.new , Todo.create ) |
UpdateView | edit + update (e.g., Todo.find(params[:id]).update ) |
DeleteView | destroy (e.g., Todo.find(params[:id]).destroy ) |
Last question- what are some other class attributes that can be set in Django? According to ChatGPT (and confirmed by the Django docs), here are some of the more useful class attributes used with ListView:
- You can define an alternative to the default result set (aka the default queryset) by using the
queryset
class attribute, i.e.queryset = Todo.objects.filter(completed=false)
. - You can set how many results per paginated page by defining the
paginate_by
attribute, i.e.paginate_by = 10
. - You can set which attribute of the queryset object will dictate the result order by using the
ordering
attribute, i.e.ordering = ["-created_at"]
.
As a last step, I ask ChatGPT to come up with a simple, "Hello World" example of using parent classes with class attributes, and inheriting from that parent class in subsequent child classes. Here's the code it gave me:
# in a file named greeter.py
class Greeter:
greeting = "Hello"
name = "World"
def say_hello(self):
print(f"{self.greeting}, {self.name}!")
class FriendlyGreeter(Greeter):
greeting = "Hi"
name = "Alice"
class FormalGreeter(Greeter):
greeting = "Good day"
name = "Mr. Smith"
greeter = Greeter()
friendlyGreeter = FriendlyGreeter()
formalGreeter = FormalGreeter()
greeter.say_hello()
friendlyGreeter.say_hello()
formalGreeter.say_hello()
When I run this code in my terminal, I get:
$ python3 greeter.py
Hello, World!
Hi, Alice!
Good day, Mr. Smith!
This result makes sense- the parent class is able to be instantiated, and when we do so we see the default values of "Hello" and "World" in-use. But when we override those values in child classes, we see the say_hello
method uses those child class attribute values instead.
Great, I answered my original question about class attributes, and several follow-up questions as well.